You can easily in php remove value from array. By using one of these PHP built-in functions like unset(), array_search(), array_keys(), array_splice() and array_diff().
I recommend you must have to know all these functions so you can use choose best and optimize method according to your algorithm.
In php remove value from array by key:
Give your index value of array into unset() function. Let’s suppose we have an array of car characteristics. If you want to remove value from the array then you have to do the below code.
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound");
unset($car_attributes[2]);
echo "<pre>";
print_r($car_attributes);
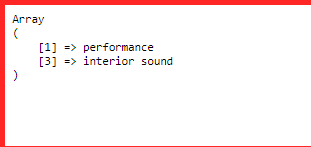
Also you can use array_splice() function in php to remove value from array by key and in output give you sorted index array.
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound");
array_splice($car_attributes, 1, 1);
echo "<pre>";
print_r($car_attributes);
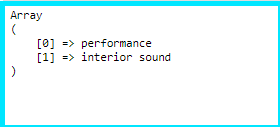
In PHP remove value from array using array_search():
Majority developer use array_search() for removing value from array.
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound");
$value = "interior sound";
if (($is_exists = array_search($value, $car_attributes)) !== false) {
unset($car_attributes[$is_exists]);
}
echo "<pre>";
print_r($car_attributes);
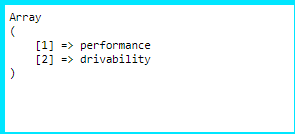
in the above code array_search() will return the first key-value then we are checking if the value exists in an array and then remove that value by using the unset() function.
Important
array_search() will work only if you have unique values in $car_attributes array. If you have duplicate values in the array then it will remove only the very first match value.
In the below code you can see we have duplicate values of “interior sound” and by using array_search() it is removing the first value.
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound", "4" => "range", "5" => "interior sound");
if (($is_exists = array_search('interior sound', $car_attributes)) !== false) {
unset($car_attributes[$is_exists]);
}
echo "<pre>";
print_r($car_attributes);
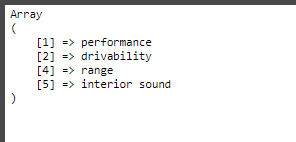
if you want to remove multiple same values then you have to use array_key() function
Remove value from array using array_keys():
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound", "4" => "range", "5" => "interior sound");
$value = "interior sound";
foreach (array_keys($car_attributes, $value) as $key) {
unset($car_attributes[$key]);
}
echo "<pre>";
print_r($car_attributes);
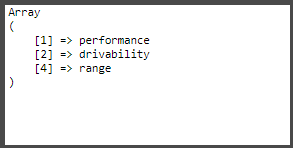
In above code we are getting matching values keys on loop and removing from array by unset function as we did above in first example.
Remove value from array using array_diff():
$car_attributes = array("1" => "performance", "2" => "drivability", "3" => "interior sound", "4" => "range", "5" => "interior sound");
$values = array("interior sound");
$car_attributes = array_diff($car_attributes, $values);
echo "<pre>";
print_r($car_attributes);
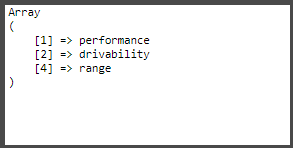
Also read JQuery Multiselect Dropdown with custom icon
How to remove specific value from array in php?
if (($is_exists = array_search(‘Your value’, $your_array)) !== false) {
unset($your_array [$is_exists]);
}
In php remove element from array by value
foreach (array_keys($array, ‘value’) as $key) {
unset($array[$key]);
}